The Most Common XSS Vulnerability in React.js Applications
If you’ve copied the renderFullPage function from the redux documentation, then you’re vulnerable to an cross site scripting attack. You most likely have an Cross Site Scripting vulnerability in your React.js application. This article shows you how to fix it in one easy step.
People are often drawn towards using React.js thanks to the benefits of isomorphic (or universal) rendering. That is, the ability to render your single page application on the server-side, send the html to the client and have the client become interactive without having to re-rendering the entire page.
Libraries like Redux even have documentation as to just how to provide this functionality. In their documentation they featured the following code snippet:
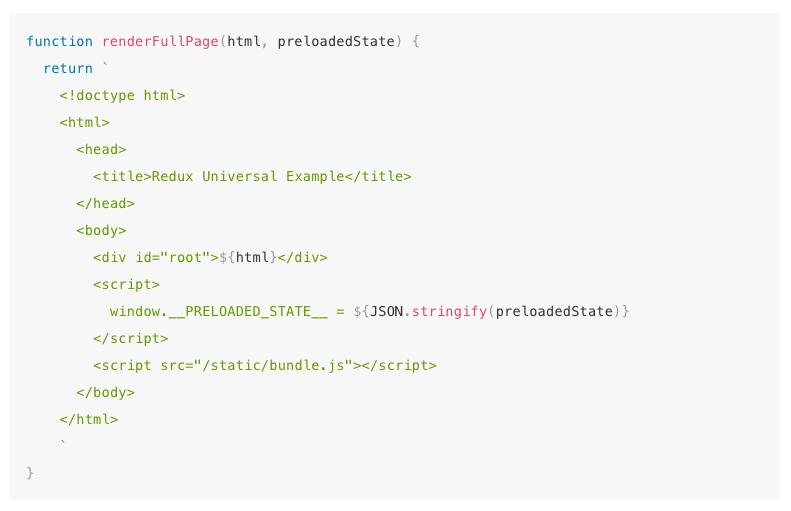
Now, you may look at that snippet and be unsure as to why there is a security issue.
Don’t worry, you’re not alone.
I recently had a conversation with Jimmy Jia in which I realised that I had accidentally exposed an application that I had worked on to an XSS Vulnerability — despite being a highly experienced software engineer with some security training.
What’s the issue, Em?
The issue with that code snippet is in how we pass the Store state into the application. In the above screenshot, we just do a JSON.stringify
call, and assign it to a global variable in a script tag. This is the vulnerability.
When a web browser parses the html of the page, and it encounters that <script>
tag, they will continue reading until they see </script>
— which means if your redux store has a value like the following in it, then when you load the client, you’ll receive an alert “You have an XSS vulnerability!”.{
user: {
username: "NodeSecurity",
bio: "as</script><script>alert('You have an XSS vulnerability!')</script>"
}
}
The browser won’t actually read until the last curly bracket, instead, it will actually finish the script tag after bio: "as
How can you prevent this XSS vulnerability?
The Open Web Application Security Project luckily has a great selection of resources about XSS prevention. To prevent this vulnerability we need a few safety measures in place in our applications:
- All user input should have HTML entities escaped. When you’re using React, it does prevent most XSS vulnerabilities, due to how it creates DOM Nodes and textual content.
- When serializing state on the server to be sent to the client, you need to serialize in a way that escapes HTML entities. This is because you’re often no longer using React to create this string, hence not having the string automatically escaped.
Engineers over at Yahoo have luckily made the latter of these safety practices very easy to implement through their Serialize JavaScript module. Which you can easily drop into any application.
First install the module with: npm install --save serialize-javascript
Then we can change the snippet from earlier to look like the following; In particular notice the change where we use serialize
instead of JSON.stringify
when assigning the value to __PRELOADED_STATE__
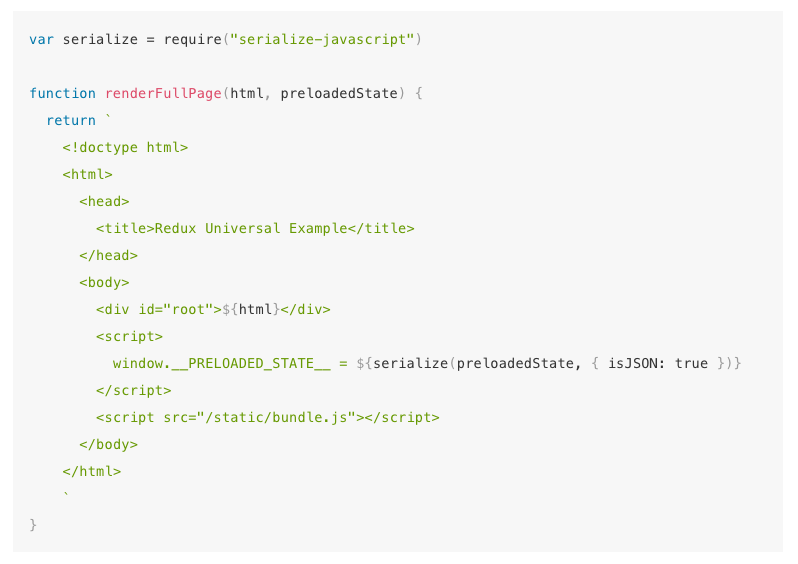
As for receiving the data on the client-side, it works just the same as before, excepting that you now just have any HTML Entities in the string escaped (they’ll look like: \\u003C\\u002Fscript\\u003E
rather than as </script>
)
Remember: Just because you’re building an application with the latest technology on the market, or technology that is used by Facebook, you still have to take care of all the standard security practices you would, if you were writing an application with a Rails, Django, PHP, or older stack.
This article has been translated into Traditional Chinese by Wendell Liu.
This post was produced with the help of a conversation with Jimmy Jia who first revealed this vulnerability to me — I had originally thought the browser worked differently and was aware of content in script tags).